一般计算机书的开头都是 Hello World
我们亦不能免俗,所以本章我们的任务就是完成最简单的 Hello World
建立目录结构
与 Python 相比,Go 对代码存放的位置还是有讲究的,毕竟这是由 Go 特殊的 package引用机制
决定的,首先建立自己存放这次代码的文件夹
1 | $ cd $GOPATH/src |
这里如果大家有Github账号,而且想上传到自己的repo的话,建议 github.com/your_user_name/repo_name 的文件夹
Hello World 应用
在 github.com/bonfy/go-mega-code 文件夹下 建立 main.go
,这是我们程序的主入口
main.go
1 | package main |
短短不到10行代码,我们的 Hello World 应用就已经完成了,而且不需要任何的其他第三方Package,只需要引入官方的 net/http 就行了,就是这么easy
让我们来运行下面的命令,看下效果
1 | $ go run main.go |
现在打开您的网络浏览器并在地址栏中输入以下URL:
1 | http://localhost:8888 |
说明
这里对上面的代码进行简单的说明
这里的 func main()
是主程序入口,主要用到了 net/http 的两个函数
1 | func HandleFunc(pattern string, handler func(ResponseWriter, *Request)) |
HandleFunc
类似于 flask的 app.route
, pattern
提供了路由路径,handler是一个函数参数,这里我们的程序中传入的是一个匿名函数, 减少了代码
ListenAndServe
第一个参数为 addr,如果不提供ip,这里只传入端口,相当于 0.0.0.0:8888
,第二个参数 Handler 传入 nil,则表示使用 Default 的 Server
另外 输出 `Hello World` 的办法,大致有三个,如下:
1 | // Case 1: w.Write byte |
其中第一种用的是 ResponseWriter 的 Write([]byte) (int, error)
方法, 而 后面两种是稍微用到了 Go 里面interface 的特性, ResponseWriter interface 要实现 Write([]byte) (int, error)
的方法,所以也就实现了 io.Writer 方法,所以可以作为 io.Writer 的类型作为 后面两个函数的参数。
这里如果想更深入的了解 net/http 处理请求的话,可以看下Go源码中的 net/http/server.go
或者看下 Go的http包详解
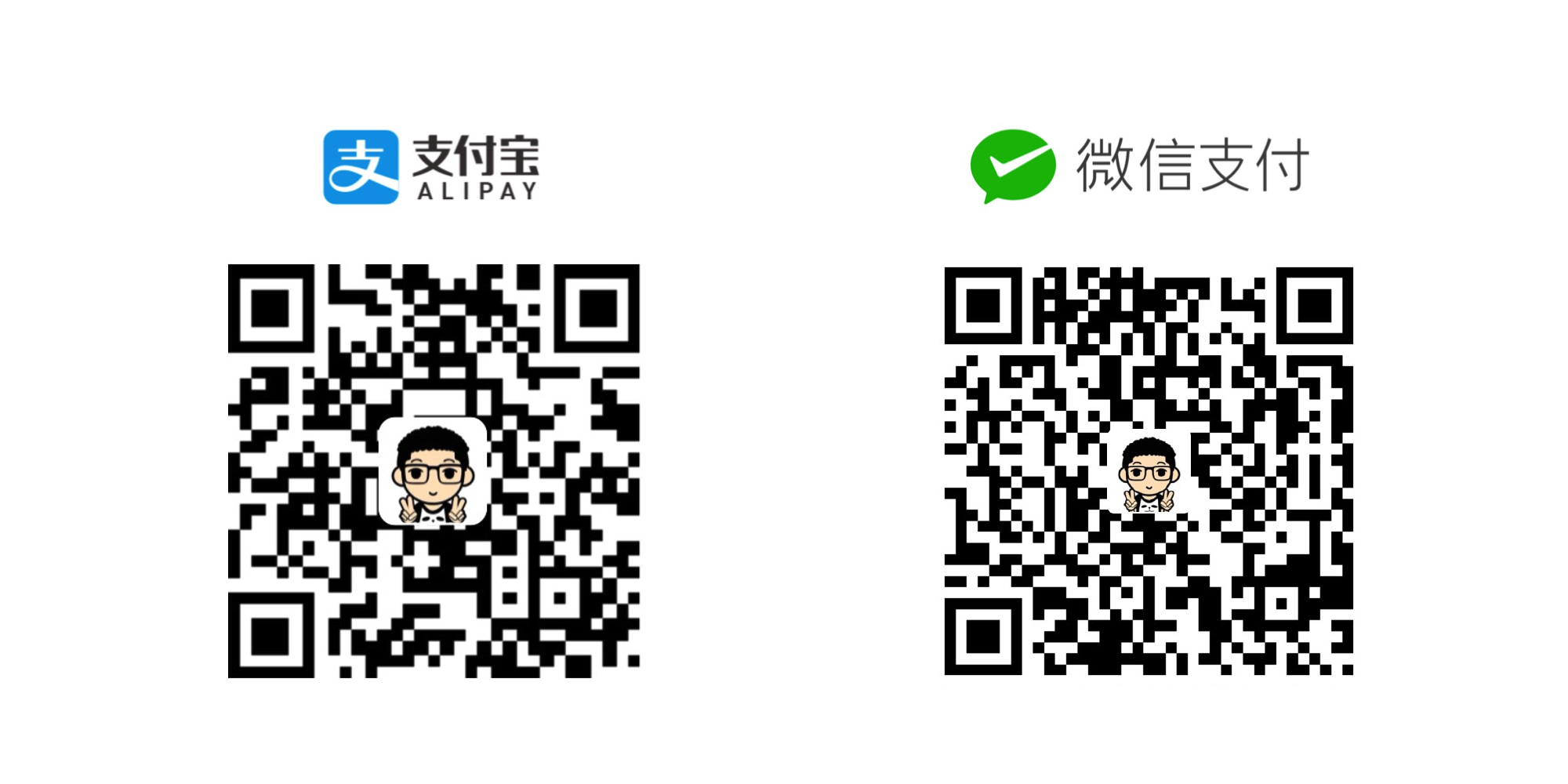
感谢鼓励